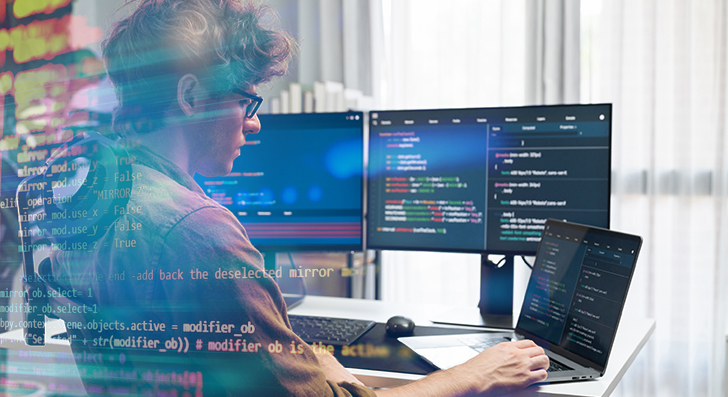
Scalability means your application can manage development—more people, far more info, and much more traffic—devoid of breaking. Like a developer, developing with scalability in your mind saves time and worry later on. Here’s a transparent and sensible guideline that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really something you bolt on later on—it should be portion of your plan from the start. Many apps fail whenever they grow rapidly because the initial design can’t tackle the extra load. For a developer, you have to Consider early regarding how your program will behave stressed.
Start by planning your architecture to become versatile. Avoid monolithic codebases in which all the things is tightly connected. As an alternative, use modular structure or microservices. These patterns break your app into smaller, independent areas. Each individual module or services can scale on its own devoid of influencing the whole program.
Also, think of your database from day a single. Will it will need to take care of one million users or perhaps a hundred? Select the suitable kind—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another critical position is to avoid hardcoding assumptions. Don’t write code that only functions below existing situations. Think of what would come about When your consumer base doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that support scaling, like message queues or party-pushed devices. These enable your application tackle extra requests devoid of finding overloaded.
Any time you Create with scalability in mind, you're not just making ready for fulfillment—you might be cutting down long run head aches. A nicely-planned procedure is simpler to keep up, adapt, and grow. It’s improved to get ready early than to rebuild afterwards.
Use the best Database
Choosing the suitable database is a vital Component of creating scalable applications. Not all databases are designed a similar, and utilizing the Incorrect you can sluggish you down or even bring about failures as your app grows.
Get started by comprehension your information. Is it remarkably structured, like rows inside of a table? If Of course, a relational database like PostgreSQL or MySQL is a great suit. These are solid with relationships, transactions, and regularity. In addition they assistance scaling procedures like read through replicas, indexing, and partitioning to handle far more traffic and knowledge.
In case your facts is more adaptable—like consumer exercise logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling massive volumes of unstructured or semi-structured data and might scale horizontally more conveniently.
Also, contemplate your browse and create designs. Are you presently carrying out numerous reads with fewer writes? Use caching and read replicas. Do you think you're managing a heavy produce load? Look into databases that will take care of high create throughput, as well as party-based information storage techniques like Apache Kafka (for momentary information streams).
It’s also wise to Consider forward. You might not have to have advanced scaling attributes now, but selecting a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your information according to your accessibility designs. And often check database efficiency when you improve.
Briefly, the appropriate databases is dependent upon your application’s construction, speed requirements, and how you expect it to grow. Consider time to pick wisely—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, every single modest delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, simple code. Stay clear of repeating logic and remove something unnecessary. Don’t select the most sophisticated solution if an easy a single works. Maintain your functions shorter, concentrated, and simple to check. Use profiling instruments to locate bottlenecks—sites the place your code requires far too extended to operate or employs too much memory.
Upcoming, check out your database queries. These generally slow points down greater than the code by itself. Make certain Just about every query only asks for the information you truly want. Stay clear of Pick out *, which fetches every thing, and as a substitute choose distinct fields. Use indexes to hurry up lookups. And stay away from executing too many joins, Specially throughout large tables.
In case you see the identical data getting asked for many times, use caching. Shop the outcome quickly using resources like Redis or Memcached therefore you don’t really have to repeat costly operations.
Also, batch your databases functions whenever you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your application much more effective.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 information could crash when they have to handle 1 million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when required. These measures aid your application remain clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more end users plus much more website traffic. If anything goes by just one server, it can promptly turn into a bottleneck. That’s where by load balancing and caching can be found in. Both of these equipment aid keep your application speedy, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server accomplishing the many work, the load balancer routes buyers to unique servers click here determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing data temporarily so it could be reused swiftly. When users ask for the identical information yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database when. It is possible to serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching lowers database load, enhances velocity, and tends to make your application more productive.
Use caching for things which don’t alter generally. And usually be sure your cache is updated when info does transform.
In brief, load balancing and caching are uncomplicated but effective instruments. Together, they help your application tackle much more end users, continue to be quick, and Recuperate from challenges. If you plan to develop, you may need both of those.
Use Cloud and Container Tools
To create scalable purposes, you'll need equipment that allow your application mature easily. That’s in which cloud platforms and containers are available in. They provide you adaptability, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t have to buy hardware or guess long term capacity. When site visitors raises, you'll be able to incorporate far more assets with just a couple clicks or routinely employing automobile-scaling. When targeted traffic drops, it is possible to scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection instruments. It is possible to target creating your app instead of managing infrastructure.
Containers are A further critical Resource. A container deals your app and everything it really should operate—code, libraries, settings—into one unit. This can make it uncomplicated to move your app involving environments, from the laptop to the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your app utilizes various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. You'll be able to update or scale parts independently, and that is great for effectiveness and reliability.
Briefly, utilizing cloud and container applications implies you could scale quickly, deploy effortlessly, and Get better rapidly when challenges occur. In order for you your app to increase without limitations, start out utilizing these instruments early. They save time, minimize possibility, and assist you to keep centered on building, not repairing.
Watch Everything
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is performing, spot concerns early, and make superior conclusions as your app grows. It’s a important Portion of making scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and in which they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes over a limit or simply a company goes down, you'll want to get notified promptly. This can help you correct concerns quick, frequently before buyers even detect.
Monitoring can also be useful after you make improvements. In case you deploy a whole new characteristic and see a spike in glitches or slowdowns, it is possible to roll it back right before it will cause actual damage.
As your application grows, site visitors and data raise. With no monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the appropriate equipment set up, you remain on top of things.
In a nutshell, checking will help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and ensuring that it works perfectly, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By designing meticulously, optimizing sensibly, and using the suitable tools, it is possible to build apps that improve smoothly without having breaking under pressure. Start off compact, Believe massive, and Establish intelligent.